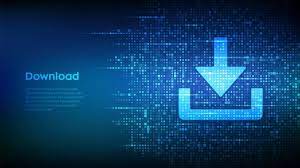
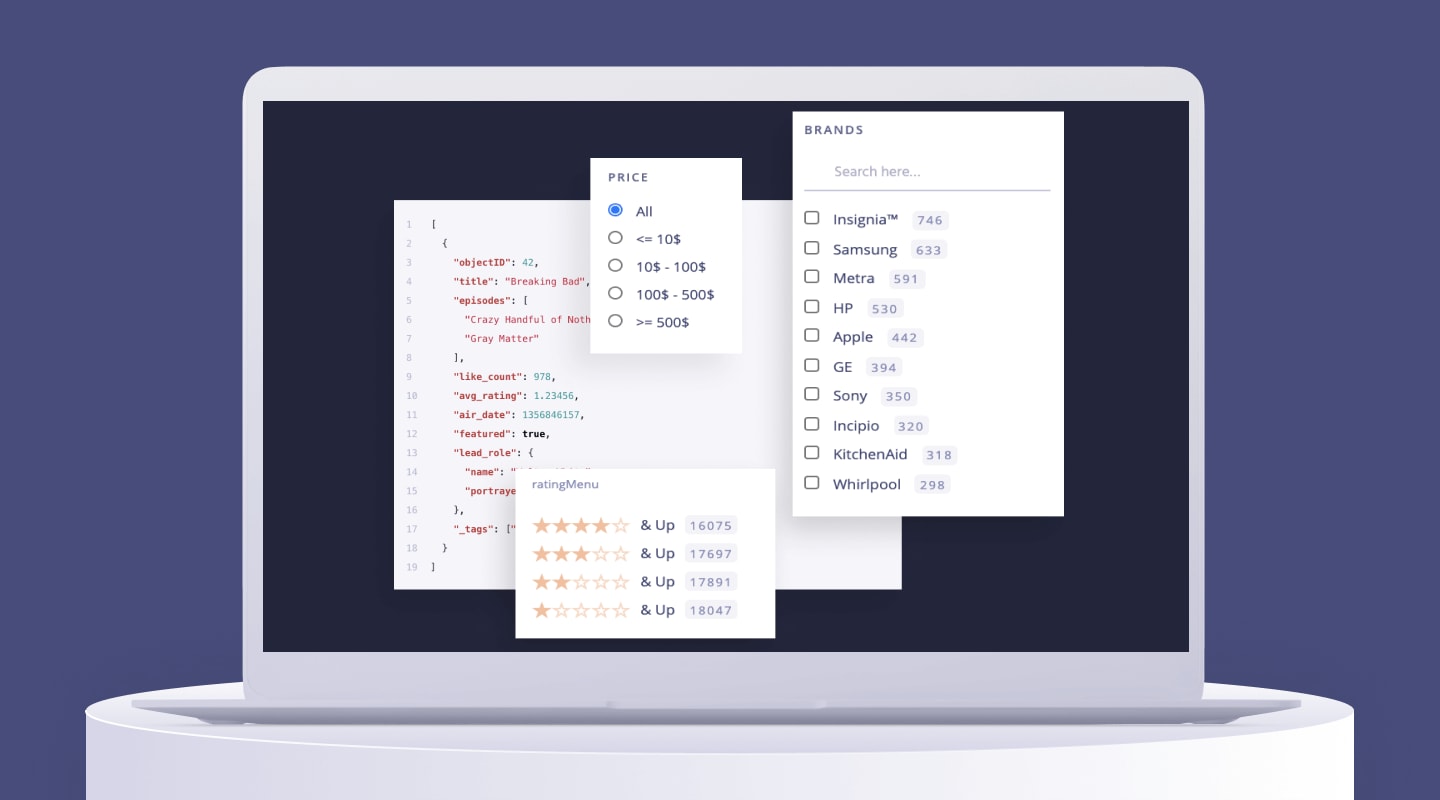
Most of the other NumPy functions which create arrays use the numpy.float64 datatype by default. We expect the datatype of A to be integers and we verify: A.dtype For example, create a 2D NumPy array from a list of lists of integers: A = np.array(,])

We can access the datatype of a NumPy array by its.
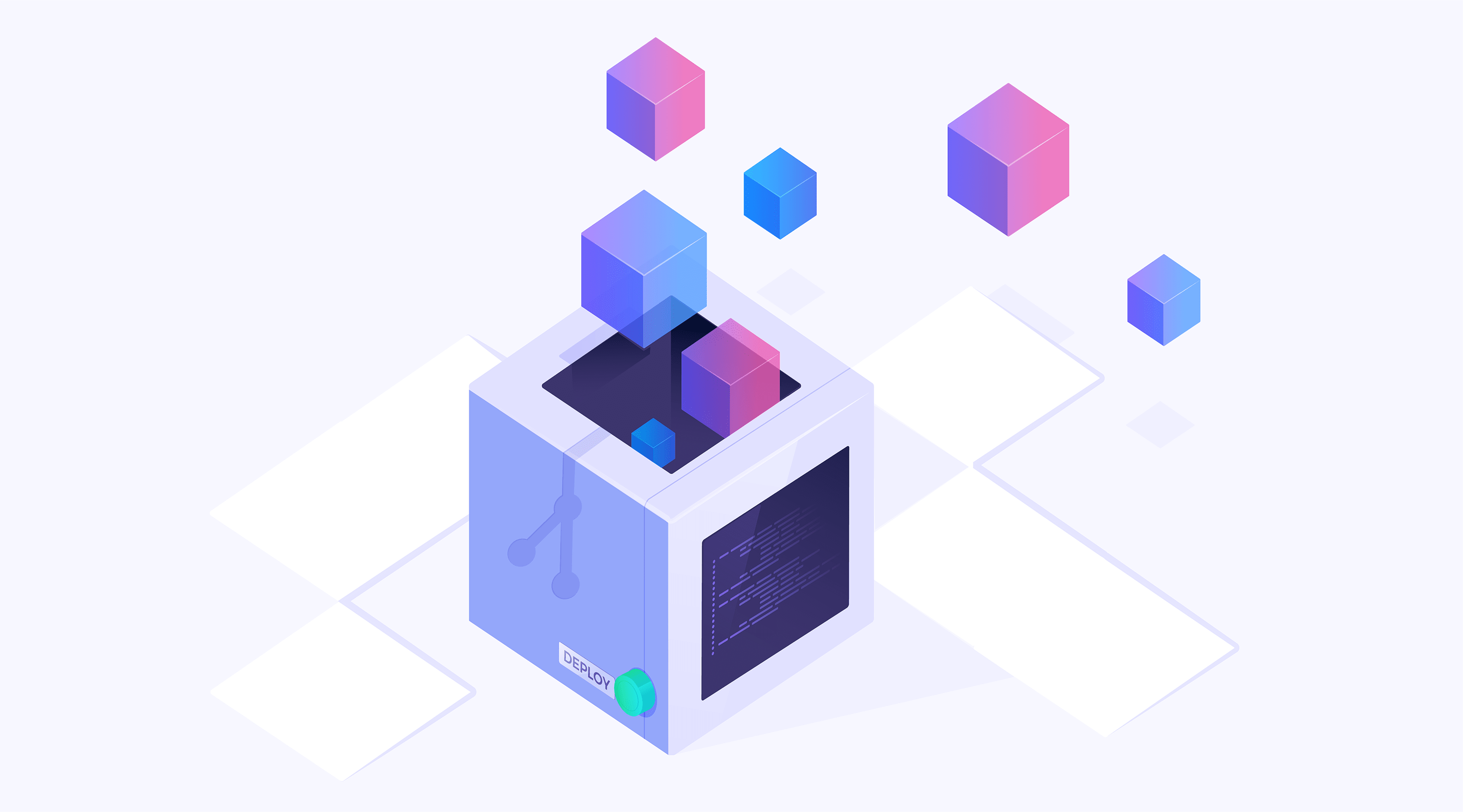
#Tsearch np.array object error how to
The most important point for now is to know how to determine if a NumPy array contains integers elements or float elements. Check out the NumPy documentation on numeric datatypes for more information. These are very similar to the built-in Python datatypes int and float but with some differences that we won't go into. There are different kinds of datatypes provided by NumPy for different applications but we'll mostly be working with the default integer type numpy.int64 and the default float type numpy.float64. We will only work with numeric arrays and our arrays will contain either integers, floats, complex numbers or booleans.
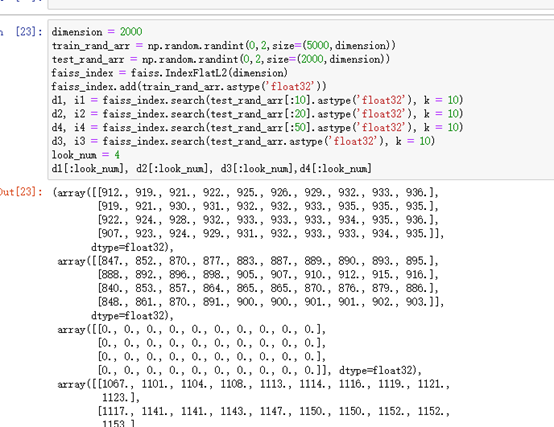
NumPy arrays are homogeneous: all entries in the array are the same datatype. The function numpy.linspace works best when we know the number of points we want in the array, and numpy.arange works best when we know step size between values in the array.Ĭreate a 1D NumPy array of zeros of length 5: z = np.zeros(5)Ĭreate a 2D NumPy array of zeros with 2 rows and 5 columns: M = np.zeros((2,5))Ĭreate a 1D NumPy array of ones of length 7: w = np.ones(7)Ĭreate a 2D NumPy array of ones with 3 rows and 2 columns: N = np.ones((3,2))Ĭreate the identity matrix of size 10: I = np.eye(10) These are the functions that we'll use most often when creating NumPy arrays. the identity matrix of size $N$)Ĭreate a 1D NumPy array with 11 equally spaced values from 0 to 1: x = np.linspace(0,1,11)Ĭreate a 1D NumPy array with values from 0 to 20 (exclusively) incremented by 2.5: y = np.arange(0,20,2.5) There are several NumPy functions for creating arrays: FunctionĬreate $n$-dimensional NumPy array from sequence aĬreate 1D NumPy array with N equally spaced values from a to b (inclusively)Ĭreate 1D NumPy array with values from a to b (exclusively) incremented by stepĬreate 1D NumPy array of zeros of length $N$Ĭreate 2D NumPy array of zeros with $n$ rows and $m$ columnsĬreate 1D NumPy array of ones of length $N$Ĭreate 2D NumPy array of ones with $n$ rows and $m$ columnsĬreate 2D NumPy array with $N$ rows and $N$ columns with ones on the diagonal (ie. For example, the following is a 3D NumPy array: N = np.array(,], ,], ,] ]) Use the built-in function type to verify the type: type(a)Ĭreate a 2D NumPy array from a Python list of lists: M = np.array(,])Ĭreate an $n$-dimensional NumPy array from nested Python lists. Notice also that a NumPy array is displayed slightly differently when output by a cell (as opposed to being explicitly printed to output by the print function): a Notice that when we print a NumPy array it looks a lot like a Python list except that the entries are separated by spaces whereas entries in a Python list are separated by commas: print() For example, create a 1D NumPy array from a Python list: a = np.array() The function numpy.array creates a NumPy array from a Python sequence such as a list, a tuple or a list of lists. See the NumPy tutorial for more about NumPy arrays. We can think of a 1D (1-dimensional) ndarray as a list, a 2D (2-dimensional) ndarray as a matrix, a 3D (3-dimensional) ndarray as a 3-tensor (or a "cube" of numbers), and so on. The fundamental object provided by the NumPy package is the ndarray. To get started with NumPy, let's adopt the standard convention and import it using the name np: import numpy as np Vectorized operations and functions which broadcast across arrays for fast computation.NumPy is the core Python package for numerical computing.
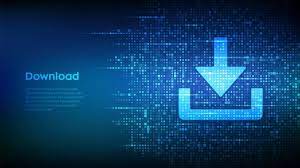